Powered by Java – Getting Started With Android Development – Part 2
What are programming languages?
Just like everyday people talk to each other in different languages, like English, French, Italian, Spanish, etc., different programming languages allow computers to communicate and pass instructions to each other to perform an action.
What is a program?
Sometimes, just one instruction doesn’t achieve the desired action. Instead, a series of instructions is needed to complete the objective. When these instructions are written as a step by step process, it’s called a program. Programs allow us to instruct a computer, process data, or convert user input into something on the screen. Internally, the computer processor exchanges electrical signals back and forth to process data.
With Java, we can write these instructions in an elegant and simple manner. Most instructions are very clear to read and understand. The computer takes care of translating a program into the electrical impulses that the processor can understand.
Programming in Java
Java is an object-oriented language, statically typed and compiled into Machine code. Let us define each of these foundational terms:
- Statically Typed: In programming, we often work with data. When a program is executed, some of the data needs to be stored, temporarily, in “containers”. We call these “containers”, variables. In statically-typed languages, we need to define the type of variable in advance. For example, if a variable is an integer, we need to define it as such, and we won’t be allowed to put something else, like a character, in it. The type of each variable and value assigned to them will be checked in the first step: compilation.
- Object-Oriented: An object-oriented language is one which is built around the reality of objects. In our day to day lives, everything around is an object. For instance, on your desk right now, you might have a pen. As an object, its name is “pen” and it has properties, like its ink color, type of ink it can hold, and its brand.
Object-oriented languages empower us to define objects and access their properties in our code. We can also send messages to objects. We can create and manipulate all sorts of objects to do different things in our applications.
Java Compilation process
Now, let’s get started with the basics. First, we must understand how the instructions we write are passed to the processor on a computer.
Briefly explained, we: 1) write code in a text editor, 2) save it in a format that the compiler understands with a “.java” file extension, 3) javac (java compiler) converts this to a “.class” format file (bytecode – class file), and 4) JVM executes the .class file on the operating system.
Always remember Java is not the base language that the operating system or a processor understands.
Source code written in Java is interpreted by the operating system using a translator known as JVM (Java Virtual Machine). JVM cannot understand the code that we write in an editor, it only understands compiled code. Hence, our need for a compiler.
The process, step-by-step, is:
- A Java compiler accepts a file with the extension .java and compiles the source code we wrote to Bytecode. This is still not the code which the processor will understand, but JVM will. A new file is created by the compiler for each .java file given to it, with a new extension, .class.
- Once the bytecode is made, the .class file is passed to the JVM, where it interprets it and converts it to Machine code.
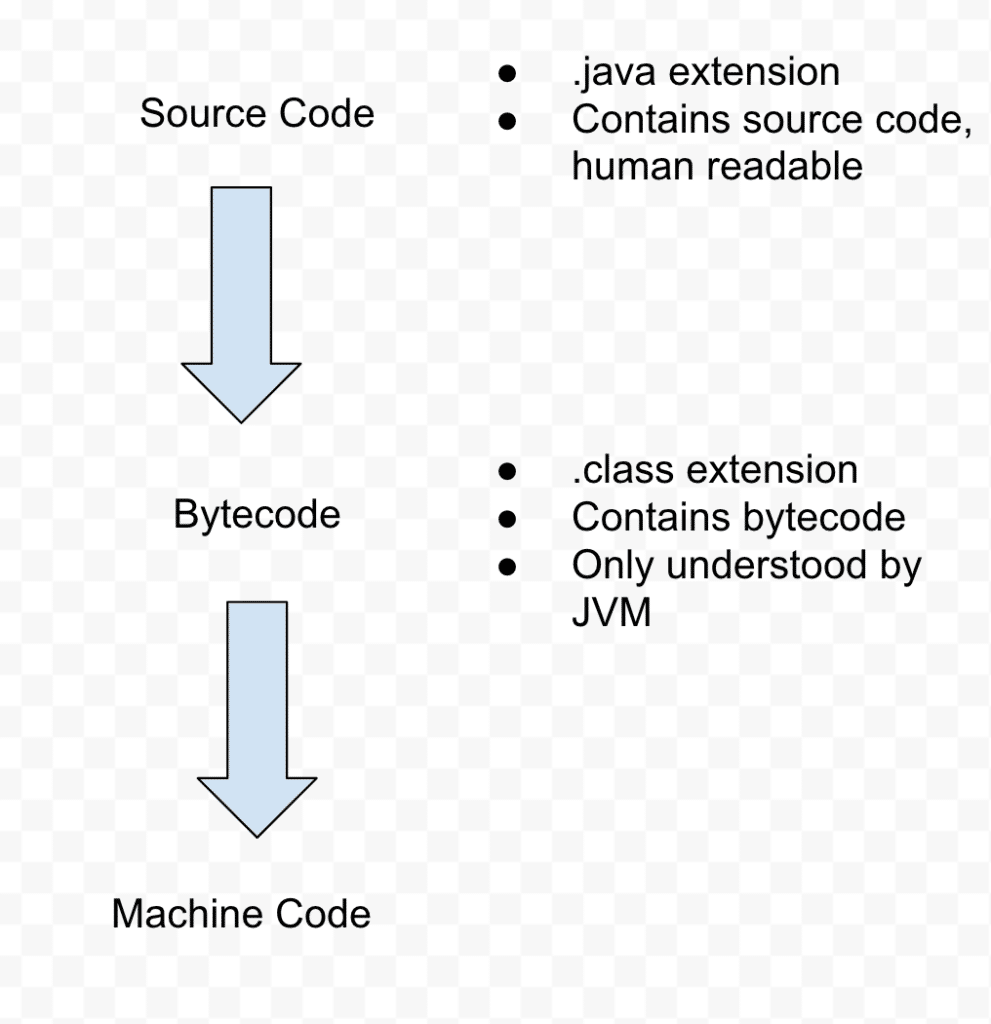
Data Types
As previously mentioned, when we define variables in Java, we must first assign a data-type to the variables, before any compilation happens. We have different use-cases to hold data. We will briefly define what data types exist in Java and what type of data they hold.
- int: An integer value, i.e. a whole number that includes positive numbers, zero and negative numbers. No decimals.
- float: A floating point holds decimal values. We should not use floats while saving data in which we need precision because the decimal place can change, or float, so data can become imprecise. When precise decimals are needed, like for currency, we should use the BigDecimal data type.
- Boolean: These are the simplest data-types, simply representing a true or false values. The Java keywords for the values are “false” and “true”, which represent 0 and 1, respectively.
- char: A single character, such as the letter ‘A’ or the symbol ‘#’. Note that lowercase and uppercase characters are different, so ‘a’ and ‘A’ are considered as two different characters. These are identified by the ASCII values.
- String: String data is a collection of characters concatenated together to make text, like “We’re learning a great language here”.
As an example, we can create a String variable, by typing:
String myName = "Paulo"; |
Here, myName is a “container” which will contain data of type String.
The first four data-types are fairly simple and straightforward. Note, the “S” in String is capital because String is a more complex (or interesting) data type. Strings are actually objects, and the naming convention in Java is that object names should start with a capital letter. An object is different than a primitive data type because it has more complex properties and methods available to it, whereas the primitive data types are limited and straightforward. For instance, the String object has a method called length() that tells us how many characters are in the String, but the float data type does not have any such method capability.
Variables
As we mentioned earlier, variables are like “containers” of data which hold a particular type of data, one that we need to declare before putting data into it. If we violate that declaration, our program will not pass the compilation stage.
String firstStr = “We’re learning a great language here”; |
Let’s break the above statement into parts and examine each part carefully.
- String This indicates what kind of data our variable will hold. This variable can only hold String types of data.
- fisrtStr Every variable in Java needs to have an identifier through which it is identified. The naming a variable follows some basic rules:
- No spaces.
- No special characters.
- It should not begin with a number.
- It can only have letters, numbers, and underscores.
- The equals sign (=) is an operator, meaning it performs a specific operation. This is an assignment operator used to assign values to variables. In this example, it is assigning the text value on the right (#4) to the variable “firstStr” on the left (#2).
- The text between the quotation marks is the String value. In Java, Strings are surrounded by quotation marks to differentiate them from a regular text used in the code.
- ; The last character in the statement is the semicolon, which is used to finish the current statement. Semicolons in Java are like periods in sentences, we use them to indicate when we’re done saying something. Every statement in Java must end with a semicolon.
Here is a simple example of how the other data types are used:
int myAge = 31; float piNum = 3.14f; short myShort = 128; boolean isEmpty = true; char lastLetterOfTitle = 'O'; |
Note the ‘f’ at the end of the float. This tells the compiler that the type is a float and not a double. Also note that char values are surrounded by single quotation marks to differentiate them from String values, which use double quotation marks.
String Concatenation
Often, we concatenate pieces of text to each other. For example:
//variables - little empty buckets //String firstName = "Paulo"; String lastName = "Dichone"; int myAge = 31; System.out.println("Hi, my name is " + lastName + " and I am " + myAge + "old.") |
Methods
Whenever we want to reuse a bunch of code, we group it together in a method. A method is like an I/O device. It takes input, does processing on the data, and provide us with an output.
Let’s examine how the length() method for a String object is used to count the number of characters in a String.
public int length() { int numberOfCharacters = 0; ... code to calculate the number of characters … return numberOfCharacters; }
Let us more closely examine each part of this method declaration:
- Line 1 declares the method with a name “length”, which is the identifier for this method. “public” defines the visibility of the method and “int” defines the type of data which this method will return to the caller. Note: If the method will run some code, but not return any kind of data, then the keyword void is used instead of a data type.
- Method names follow roughly the same rules as variable names: letters, numbers, and underscores, but they cannot start with a number.
- After the name of the method, we see a set of empty parentheses. The required empty parentheses indicates that we do not pass any data or variables into the method when we call it.
- Line 1 ends with an opening curly brace, its matching closing curly brace is on line 5. In Java, blocks of code, like the code that make up a method, are often surrounded by curly braces to designate the code that should be run. This block of code belongs to the “length” method.
- Line 4 includes the “return” statement. It signifies what data this method has processed and is returning. The int variable is returned. That is, the actual number stored in the variable will be the return value of the length() method.
Calling a method
To use the method we defined above, we need to call it with an object.
String firstStr = “We’re learning a great language here”; int textLength = firstStr.length();
We have a String which has 36 characters. We call the method on the next line using dot notation, which places a period after a Class or variable name. This dot tells the method, which object it should check.
We store the returned value in a new int variable named textLength.
Why use methods?
Methods are powerful tools because they are reusable. Suppose we want to find the length of ten different strings. We won’t have to write the logic to find the length of the String ten different times. Instead, we will just call our method with different String objects.
Comments
Many times, we need to remember what our code does and how the logic works the way it does. We have the ability to add notes to our code and/or mention the algorithm we used to solve the problem, using Comments. Comments in Java are lines of code that do not affect how the program runs.
Single Line Comments
Single-line comments begin with two forward slashes: //. Everything on the line after the forward slashes makes up the comment and is completely ignored by the program.
// This is a single-line comment String text = "Something comes here."; // Only this part is a commen
t |
Multi Line Comments
If we want longer comments that cover more than one line, we use an opening marker to mark the start of the comment, /*, and a closing marker to mark the end of the comment, */.
/* This is a multi-line comment. Though only the opening * and closing markers are required, we often add an * asterisk at the beginning of each line to make it * more readable, and end the comment with the ending * marker on a new line. */
Leave your comments below. See you next time.